Being called solid is a compliment. The word carries the positive notions of reliability and respectability. All of us should aim to become one.
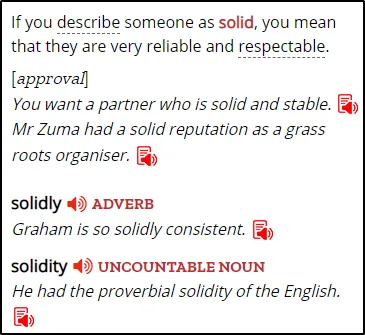
https://www.collinsdictionary.com/dictionary/english/solid (screenshot)
If you are a software engineer or want to become one, you are in luck because there is a set of SOLID principles to guide your way.
SOLID is an acronym in the software engineering profession. It stands for:
- Single Responsibility principle
- Open-closed principle
- Liskov Substitution principle
- Interface segregation principle
- Dependency inversion principle
The term was coined by Michael Feather around 2004 for the 5 object-oriented design principles first collected by Uncle Bob in his 2000 paper Design Principles and Design Patterns and his other articles and books
Let’s look at these principles in more detail.
Single Responsibility
Each class should have a single responsibility.
An EmailService
should just concern itself with sending emails, not with sending emails and updating order status.
A Car
class should be about cars only, not other types of motorized vehicles. If we need to describe an electric car, then a subclass such asElectricCar
is more appropriate than overloading Car
with electric and petrol car characteristics.
Open-close principle
A module should be open for extension but closed for modification
The EmailService
can be extended by a RecallableEmailService
which adds recall-ability feature to the email just sent.
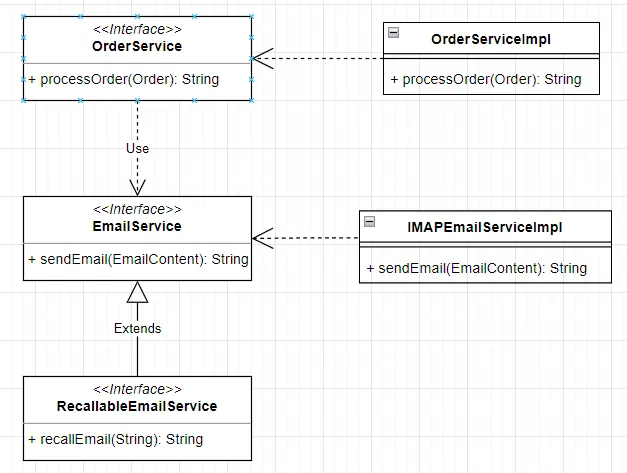
EmailService and its associations
The ElectricCar
adds electric features without modifying the internal of Car
.
Liskov substitution
Subclasses should be substitutable for their base classes
In the context of Object-oriented design, a class or method that accepts an instance of a base class should be able to take any instance of its subclass.
An OrderService
should be able to use any instance that conforms to the interface of EmailService
without concerning that the instance’s actual class is EmailService
itself or RecallableEmailService
An UrbanTripPlanner
should be able to use any instance of Car
to determine a route without knowing whether it represents an electric or petrol car.
Interface segregation
Many client specific interfaces are better than one general purpose interface
An OrderService
does not need to recallEmail(...)
. Hence it only depends on EmailService
for sending emails, not RecallableEmailService
Similarly, an UrbanTripPlanner
does not need the recharge(...)
method from an EletricCar
. It depends only on the interface provided by Car
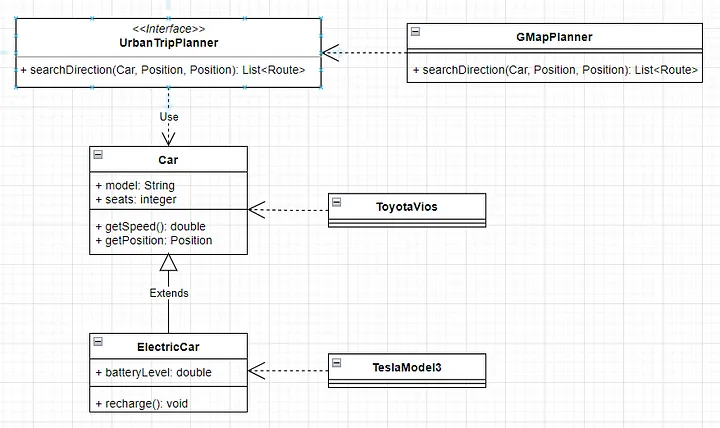
Car and its associations
Dependency inversion
Depend upon Abstractions. Do not depend upon concretions.
The procedural dependency mechanism starts from the top, gradually expands, and depends on concrete implementation details.
In Object-oriented programming, the dependency is inverted. The majority of dependencies should be upon abstractions. And where implementation exists, its relationship with abstraction inverts. Rather than being directly depended on, the implementation now depends on abstraction.
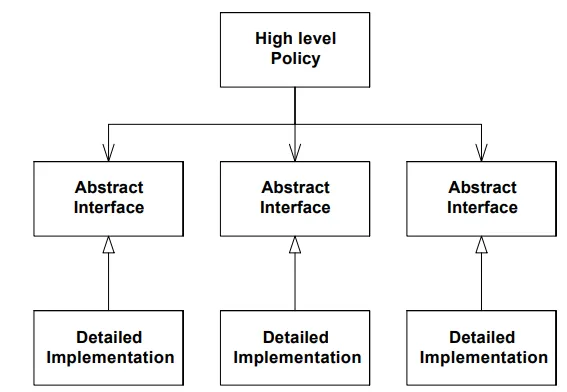
Source: Uncle Bob’s Design Principles and Design Patterns
An OrderService
depends on the interface provided by EmailService
and not its IMAP or POP implementation.
An UrbanTripPlanner
depends on the interface provided by Car
, not its Tesla or Toyota implementation
Conclusion
We have examined the origin of the SOLID acronym, as well as looked at each of them in detail with 2 object-oriented design use cases each.
You may have followed some of these principles in the past without knowing because they are good practices. Encapsulation, in my opinion, is following the Open-close principle.
You may have also applied some of them because it is the way things are. Syntactically, an ElectricCar
object can always pass in for a Car
parameter, thereby following the Liskov substitution principle. If you have used Dependency Injection, you already follow the Dependency Inversion principle.
Other principles require a bit of thought. The Single Responsibility principle needs a good OO model to keep the method/class/module clean and lean. You may need good judgment to determine how deep the segregation should be while following the Interface Segregation principle.
References
- Design Principles and Design Patterns paper
- Principles of OOD article
- Clean Architecture: A Craftsman’s Guide to Software Structure and Design
- https://en.wikipedia.org/wiki/SOLID
If you like this article, please follow me for more quality content.
Other articles in this series:
- Becoming a SOLID developer
- SOLID in Action — the Single Responsibility Principle
- SOLID in Action — the Open-closed principle
- SOLID in Action — the Liskov Substitution principle
- SOLID in Action — the Interface Segregation principle
- SOLID in Action — the Dependency Inversion principle
Thank you.